Arbitrum Nova can reuse most Ethereum Development tools and libraries
Arbitrum Nova is an improvement over the earlier version of Arbitrum called Arbitrum One, as it provides faster transaction processing times and lower gas fees. It uses a Rollup architecture that allows for secure and efficient batch processing of transactions while maintaining the same level of security as the Ethereum mainnet.
Nova can be used for users or projects with large transaction volumes, such as game developers, social projects, and blockchain applications like NFTs, & DeFi. The Arbitrum Nova introduced the AnyTurst technology to lower the cost even further by accepting a mild-trust assumption.
AnyTrust relies instead on an external Data Availability Committee (hereafter, "the Committee") to store data and provide it on demand. The Committee has N members, of which AnyTrust assumes at least two are honest. This means that if N-1 Committee members promise to provide access to some data, at least one of the promising parties must be honest, ensuring that the data will be available so that the Rollup protocol can function correctly. Arbitrum Nova is fully committed to being compatible with the EVM so that you can use the familiar development tools and programming languages you do on the EVM Layer1.
Smart contracts are programming logic that is executed automatically when certain condition(s) are met. In the Arbitrum Nova, smart contracts can be written in the Solidity programming language.
Please refer to the official documentation of Solidity for any queries.
IDE and Libraries
Multiple IDEs and libraries can be used for developing and deploying smart contracts.
- Remix IDE: a powerful open-source tool that helps you write Solidity contracts straight from the browser. It is written in JavaScript and supports both usages in the browser, but runs locally and in a desktop version. Remix IDE has modules for testing, debugging, deploying smart contracts, and much more.
- Truffle: development tool that allows users to compile, test, debug, and deploy smart contracts.
- Web3JS: a library collection that allows you to interact with a local or remote EVM node using HTTP, IPC, or WebSocket.
Steps of Smart Contract Deployment
- Write the Smart Contract Code: The first step is to write the smart contract code in a high-level programming language like Solidity, Vyper, or others. This code will be compiled into bytecode that can be executed by the network.
- Compile the Smart Contract Code: Once you have written the smart contract code, you need to compile it using a compiler such as the Solidity compiler. The compiler will produce the bytecode that can be deployed to the network.
- Connect to an Arbitrum Nova Network: To deploy the smart contract, you need to connect to an Arbitrum Nova network.
- Create a Wallet: You need to create a wallet that will be used to deploy the smart contract to the Arbitrum Nova network. You can use a wallet such as Metamask, MyEtherWallet, or others.
- Deploy the Smart Contract: Once you have connected to an Arbitrum Nova network and created a wallet, you can deploy the smart contract using a tool such as Remix, Truffle, or others. You will need to specify the bytecode of the smart contract and the gas limit for the deployment. The deployment will create a transaction on the Arbitrum Nova network that will be broadcast to the network for validation and inclusion in a block.
- Verify the Smart Contract: After deploying the smart contract, you may want to verify its source code on a blockchain explorer. This will allow others to see the source code of the smart contract and verify that it matches the deployed bytecode.
Deploy Sample Contract to Arbitrum Nova Chain
Below is an example of a smart contract that allows users to set and retrieve messages.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SampleContract {
string public message;
constructor(string memory _message) {
message = _message;
}
function setMessage(string memory _newMessage) public {
message = _newMessage;
}
}
This contract creates a simple storage for a message that can be set and retrieved. The message variable is declared as public, which means that it can be read by anyone. The setMessage function allows anyone to update the message variable.
The constructor function sets the initial value of the message variable when the contract is deployed to the Arbitrum Nova network. The memory keyword indicates that the argument is stored in memory, rather than in storage on the blockchain.
To deploy this contract, you must compile the code into bytecode and then deploy it to the Arbitrum Nova network using a tool such as Remix or Truffle. Once deployed, you could interact with the contract using a web3 provider such as MetaMask or a blockchain explorer such as Etherscan.
Step 1: Go to the Remix IDE, and create the Message.sol file and copy the example to the file.
Step 2: Compile the Solidity code.
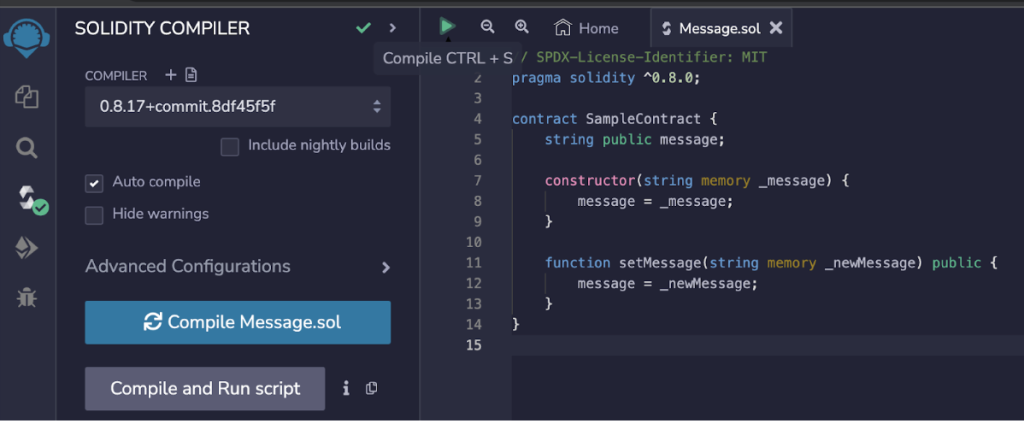
Step 3: Configure your Metamask with the network info provided. Go to Metamask Settings and Add a Network. The NodeReal RPC URL you can find in your API marketplace.
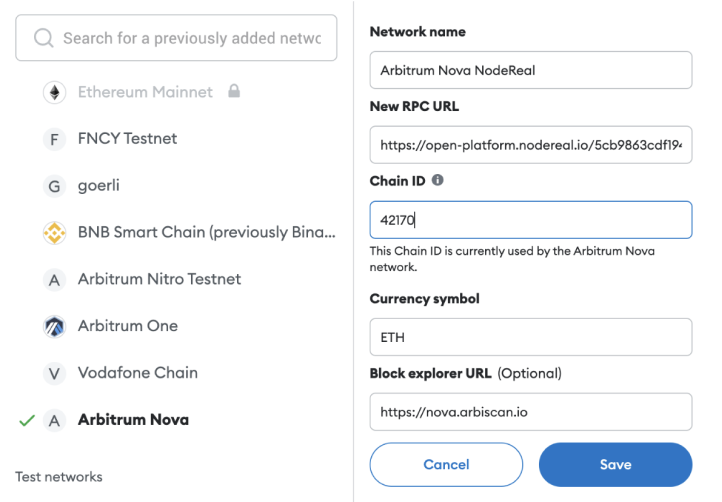
Step 4: Deposit your ETH to the Arbitrum Nova through the Arbitrum Nova Bridge.
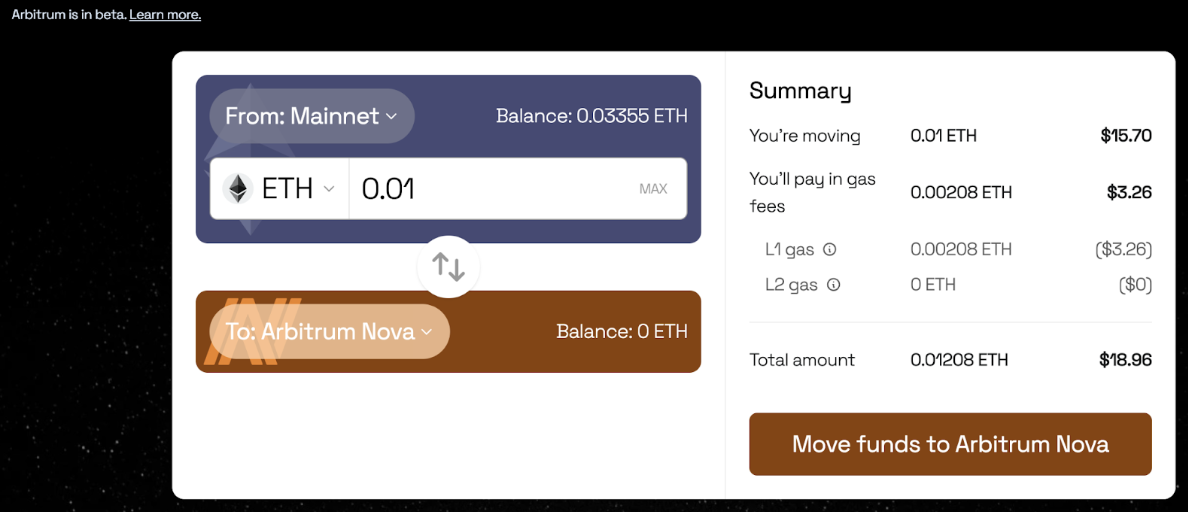
And you need to wait for a while(~15mins) to get your transaction confirmation on Layer 2.
Step 5: Switch your wallet to Arbitrum Nova to check your balance after the waiting time.
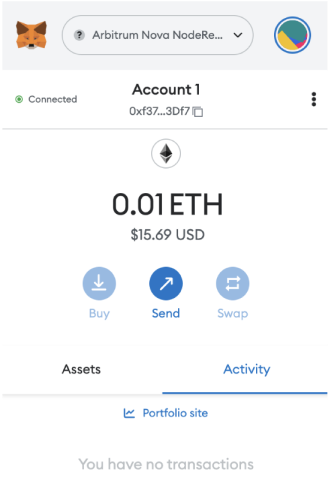
Step 6: Configure your wallet in Remix.
Please note the environment needed to select the “Injected Provider-MetaMask”, enter your public address, and click deploy.
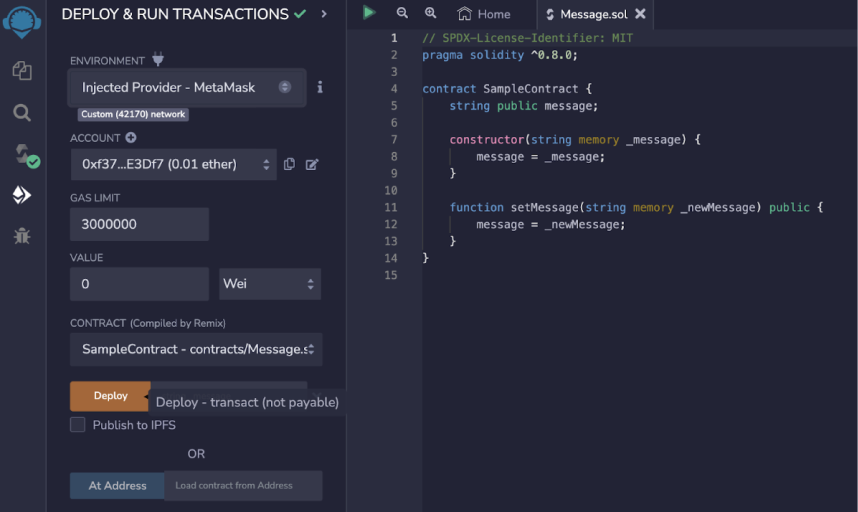
At the same time, your wallet will notify you to confirm the transaction to deploy the contract and click confirm to continue.
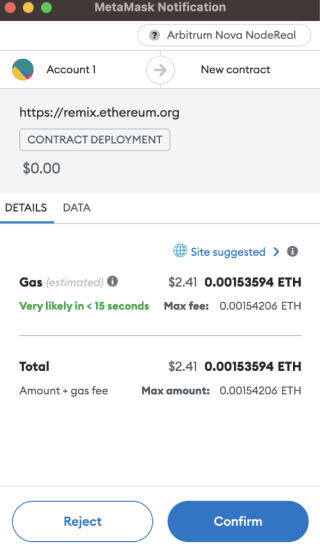
Step 7: Test the deployed contract.
In the deployed contracts section, enter the message you want to send. For example, “Hello Arbitrum Nova” and click send Message. You need to confirm the transaction on your wallet again to pay for the gas fee.

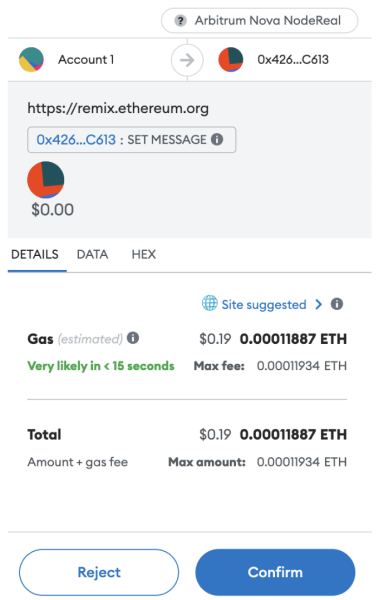
Step 8: Retrieve the message.
In the “Deployed Contracts” section, click the message to retrieve.
Step 9: You can also view the transactions through the Arbitrum Nova Block Explorer.
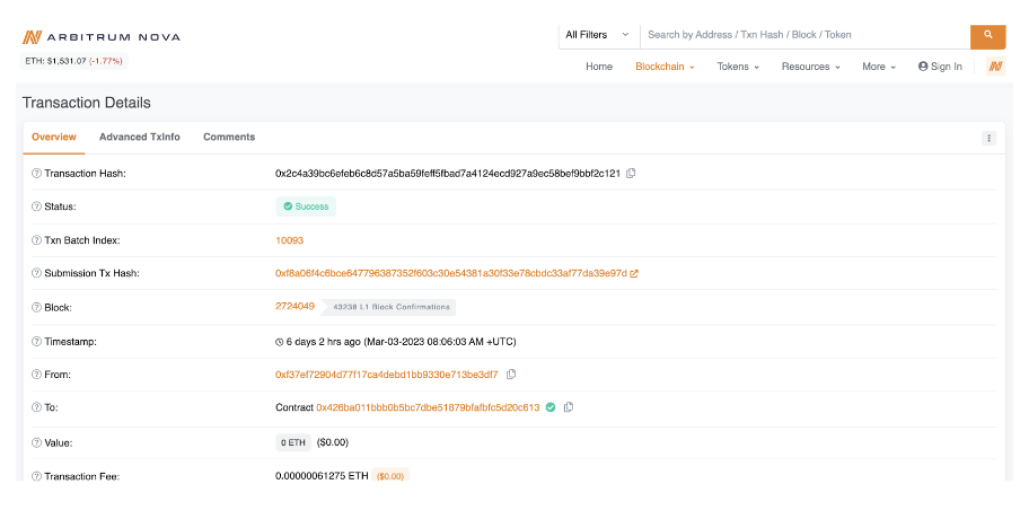
About NodeReal
NodeReal is a one-stop blockchain infrastructure and service provider that embraces the high-speed blockchain era and empowers developers by “Make your Web3 Real”. We provide scalable, reliable, and efficient blockchain solutions for everyone, aiming to support the adoption, growth, and long-term success of the Web3 ecosystem.
Join Our Community
Join our community to learn more about NodeReal and stay up to date!